
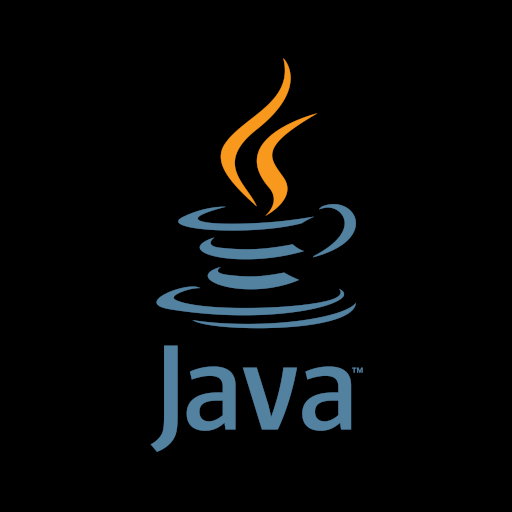
I had never heard of Phaser, but it looks pretty cool. I just read Baeldung’s Guide to Phaser and correct me if I’m wrong, but doesn’t it kind of seem like a race condition (it could just be how they use it in the examples)?
class LongRunningAction implements Runnable {
private String threadName;
private Phaser ph;
LongRunningAction(String threadName, Phaser ph) {
this.threadName = threadName;
this.ph = ph;
ph.register();
}
@Override
public void run() {
ph.arriveAndAwaitAdvance();
try {
Thread.sleep(20);
} catch (InterruptedException e) {
e.printStackTrace();
}
ph.arriveAndDeregister();
}
}
then
executorService.submit(new LongRunningAction("thread-1", ph));
executorService.submit(new LongRunningAction("thread-2", ph));
executorService.submit(new LongRunningAction("thread-3", ph));
if ph.arriveAndAwaitAdvance();
is called before all of the LongRunningAction
s are initialized, won’t it proceed before it is supposed to?
Optional has more syntactic sugar for more complex scenarios / functional call chaining that prevents repetitive
if
checksOptional.ofNullable(myObj) .map(MyClass::getProperty) .map(MyOtherClass::getAnotherProperty) .filter(obj -> somePredicate(obj)) .orElse(null)
This is completely null safe, the function calls are only made if the object is not null