
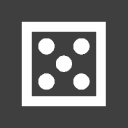
So I’m trying to create a javascript that will scan the User’s description/role for the following fields: Last_Name, Race, Political Entity, Rank, Position to be used in Character description/personality/instruction/role and Initial chat messages, But it wouldn’t work. So I came up with a twork around where its stored in the javascript. I require help in getting a javascript code that scans User’s description/role.
Original
function extractInfo(description) {
const fields = {
lastName: description.split('Last Name:')[1].split('\n')[0].trim(),
race: description.split('Race:')[1].split('\n')[0].trim(),
politicalEntity: description.split('Political Entity:')[1].split('\n')[0].trim(),
rank: description.split('Rank:')[1].split('\n')[0].trim(),
position: description.split('Position:')[1].split('\n')[0].trim()
};
return {
getLastName: () => fields.lastName,
getRace: () => fields.race,
getPoliticalEntity: () => fields.politicalEntity,
getRank: () => fields.rank,
getPosition: () => fields.position
};
}
const info = extractInfo(user.description);
console.log(info.getLastName()); // Should output the last name
console.log(info.getRace()); // Should output the race
console.log(info.getPoliticalEntity()); // Should output the political entity
console.log(info.getRank()); // Should output the rank
console.log(info.getPosition()); // Should output the position
Retrieve each field by calling info.getLastName()
, info.getRace(),
and so on. Problem is replacing user.description
with the actual string containing the user’s description.
Work Around
function extractInfo(description) {
const namePattern = /(?:(?:\b\w+\s){2,})(?:\b\w+\b)/;
const lastName = description.match(namePattern)[1];
const racePattern = /\bRace\b\s*:\s*([\w\s]+)/;
const race = description.match(racePattern)?.length > 0 ? description.match(racePattern)[1] : "Unknown";
const politicalEntityPattern = /\bPolitical Entity\b\s*:\s*([\w\s]+)/;
const politicalEntity = description.match(politicalEntityPattern)?.length > 0 ? description.match(politicalEntityPattern)[1] : "Unknown";
const rankPattern = /\bRank\b\s*:\s*([\w\s]+)/;
const rank = description.match(rankPattern)?.length > 0 ? description.match(rankPattern)[1] : "Unknown";
const positionPattern = /\bPosition\b\s*:\s*([\w\s]+)/;
const position = description.match(positionPattern)?.length > 0 ? description.match(positionPattern)[1] : "Unknown";
return {
lastName: <lastName>,
race: <race>,
politicalEntity: <politicalEntity,>
rank: <rank>,
position: <position>
};
}
const updatedDescription = "<short description>";
const updatedResult = extractInfo(updatedDescription);
console.log(updatedResult);
Where you can call each field individually by accessing the properties of the returned object, like result.lastName
, result.race
, result.politicalEntity
, and result.rank.
As a general strategy for parsing stuff out of free-form/natural language, I’d definitely recommend using the AI text generation rather than regex, since natural language is so diverse that regex will fail a lot of the time. In most cases there are too many different ways that things can be said to be able to capture them all with regex.
I assume you’re talking about perchance.org/ai-character-chat custom code, and if so, you could do something like this:
More info on custom code for perchance.org/ai-character-chat is here: https://rentry.org/82hwif
Does not seem to work from the Custom JavaScript code field, if I put into the chatbox then it works after words except of course Initial chat messages